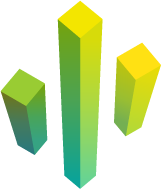
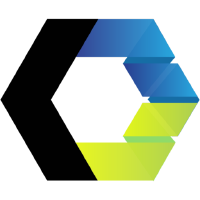

Needle Engine as Web Component
Needle Engine provides an easy-to-use web component that can be used to display rich, interactive 3D scenes directly in HTML with just a few lines of code. It's the same web component that powers our integrations.
Once you outgrow the configuration options of the web component, you can extend it with custom scripts and components, and full programmatic scene graph access.
Use the integrations!
For complex 3D scenes and fast iteration, we recommend using Needle Engine with one of our integrations. They provide a powerful creation workflow, including a live preview, hot reloading, and an advanced build pipeline with 3D optimizations.
Quick Start
<!DOCTYPE html>
<html>
<head>
<!-- Import the Needle Engine module -->
<script
type="module"
src="https://unpkg.com/@needle-tools/engine/dist/needle-engine.min.js">
</script>
</head>
<body style="margin:0; padding:0;">
<!--
Add the <needle-engine> HTML component to your page, and specify a source file.
This .glb file contains interactions, sounds, a skybox, and animations,
because it was exported from our Unity integration.
-->
<needle-engine src="https://cloud.needle.tools/api/v1/public/873a48a/10801b111/MusicalInstrument.glb" background-blurriness="0.8"></needle-engine>
</body>
</html>
Open this example on Stackblitz
Install from npm
You can work directly with Needle Engine without using any Integration. Needle Engine uses three.js as scene graph and rendering library, so all functionality from three.js is available in Needle as well.
You can install Needle Engine from npm
by running:npm i @needle-tools/engine
Install needle-engine from a CDN
While our default template uses vite, Needle Engine can also be used directly with vanilla Javascript – without using any bundler.
You can add a complete, prebundled version of Needle Engine to your website with just a line of code. This includes our core components, physics, particles, networking, XR, and more. Use this if you're not sure!
<script type="module"
src="https://unpkg.com/@needle-tools/engine/dist/needle-engine.min.js">
</script>
If you know your project doesn't require physics features, you can also use a smaller version of Needle Engine, without the physics engine. This will reduce the total downloaded size.
<script type="module"
src="https://unpkg.com/@needle-tools/engine/dist/needle-engine.light.min.js">
</script>
Many examples can be found on StackBlitz.
Rapid Prototyping on StackBlitz
For quick experiments, we provide a convenient link to create a new project ready to start: engine.needle.tools/new
Local Development with VS Code
If you want to work with Needle Engine without any integration, then you'll likely want to run a local server for your website. Here's how you can do that with Visual Studio Code:
- Open the folder with your HTML file in Visual Studio Code.
- Install the LiveServer extension.
- Activate live-server (there's a button "Go Live" in the footer of VSCode)
- Open
http://localhost:5500/index.html
in your web browser, if it doesn't open automatically.
three.js and Needle Engine
Since Needle Engine uses three.js as scene graph and rendering library, all functionality from three.js is available in Needle as well and can be used from component scripts. We're using a fork of three.js that includes additional features and improvements, especially in relation to WebXR, Animation, and USDZ export.
Tips
Make sure to update the <needle-engine src="myScene.glb">
path to an existing glb file or download this sample glb and put it in the same folder as the index.html, name it myScene.glb
or update the src path.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<link rel="icon" href="favicon.ico">
<meta name="viewport" content="width=device-width, user-scalable=no">
<title>Made with Needle</title>
<!-- importmap -->
<script type="importmap">
{
"imports": {
"three": "https://unpkg.com/three/build/three.module.js",
"three/": "https://unpkg.com/three/"
}
}
</script>
<!-- parcel require must currently defined somewhere for peerjs -->
<script> var parcelRequire; </script>
<!-- the .light version does not include dependencies like Rapier.js (so no physics) to reduce the bundle size, if your project needs physics then just change it to needle-engine.min.js -->
<script type="module" src="https://unpkg.com/@needle-tools/[email protected]/dist/needle-engine.light.min.js"></script>
<style>
body { margin: 0; }
needle-engine {
position: absolute;
display: block;
width: 100%;
height: 100%;
background: grey;
}
</style>
</head>
<body>
<!-- load a gltf or glb here as your scene and listen to the finished event to start interacting with it -->
<needle-engine src="myScene.glb" loadfinished="onLoaded"></needle-engine>
</body>
<script>
function onLoaded(ctx) {
console.log("Loading a glb file finished 😎");
console.log("This is the scene: ", ctx.scene);
}
</script>
</html>